在Java中,Object
类是所有类的超类,有12大方法。
public class Object { public Object() {} public final native Class<?> getClass(); public native int hashCode(); public boolean equals(Object obj); protected native Object clone() throws CloneNotSupportedException; public String toString(); public final native void notify(); public final native void notifyAll(); public final native void wait(long timeout) throws InterruptedException; public final void wait(long timeout, int nanos) throws InterruptedException; public final void wait() throws InterruptedException; protected void finalize() throws Throwable { } }
equals(Object obj)
比较两个对象是否相等,使用引用比较,只有当两个对象的引用相同时才返回true
。
Object obj1 = new Object(); Object obj2 = new Object(); Object obj3 = obj1; System.out.println(obj1.equals(obj2)); // false System.out.println(obj1.equals(obj3)); // true
但是,许多类会重写这个方法,以提供基于内容的比较。
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } MyClass other = (MyClass) obj; return Objects.equals(this.someField, other.someField); }
hashCode()
返回对象的哈希码,返回一个本地方法生成的整数,通常与对象的内存地址有关。
例如:HashMap
,就会使用到hashcode来定位数据存储位置。
当重写equals
方法时,也应重写hashCode
方法,以确保相等的对象具有相等的哈希码。
@Override public int hashCode() { return Objects.hash(someField); }
clone()
创建并返回当前对象的一个副本,需要实现Cloneable
接口,默认实现:创建对象的浅表副本。
class MyClass implements Cloneable { @Override protected Object clone() throws CloneNotSupportedException { return super.clone(); } }
finalize()
在垃圾回收器回收对象之前调用,用于清理资源。
@Override protected void finalize() throws Throwable { try { // 清理资源 } finally { super.finalize(); } }
getClass()
返回对象的运行时类。
Object obj = new Object(); System.out.println(obj.getClass()); // class java.lang.Object
notify()
, notifyAll()
, wait()
notify()
:唤醒一个正在等待该对象监视器的线程。
notifyAll()
:唤醒所有正在等待该对象监视器的线程。
wait()
:使当前线程等待,直到其他线程调用notify()
或notifyAll()
。
synchronized(obj) { obj.wait(); } synchronized(obj) { obj.notify(); }
wait(long timeout)
, wait(long timeout, int nanos)
使当前线程等待指定时间或直到被唤醒。
synchronized(obj) { obj.wait(1000); // 等待1秒 }
mikechen
mikechen睿哥,10年+大厂架构经验,资深技术专家,就职于阿里巴巴、淘宝、百度等一线互联网大厂。
关注「mikechen」公众号,获取更多技术干货!
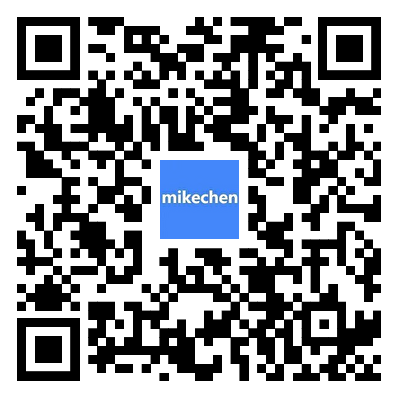
后台回复【架构】即可获取《阿里架构师进阶专题全部合集》,后台回复【面试】即可获取《史上最全阿里Java面试题总结》